Hi Himanshu, this is a great topic, I know too late, but I was able to use the Kore.AI API to automatically get the list of all the questions on the bot.
The first point is to implement a proper JWT mechanism. In my case, I 've updated the code provided by Kore with a second function. If you call this API with /app you will get the JWT token without the timestamp (required for the API).
router.post(’/app’, function(req, res, next) {
console.log(req.body);
var clientSecret = req.body.clientSecret;
var options = {
“appId”:req.body.appId};
console.log(options);
var token = jwt.sign(options, clientSecret,{noTimestamp:true});
res.send({“jwt”:token});
});
Then, you can design a dialog that looks like the following:
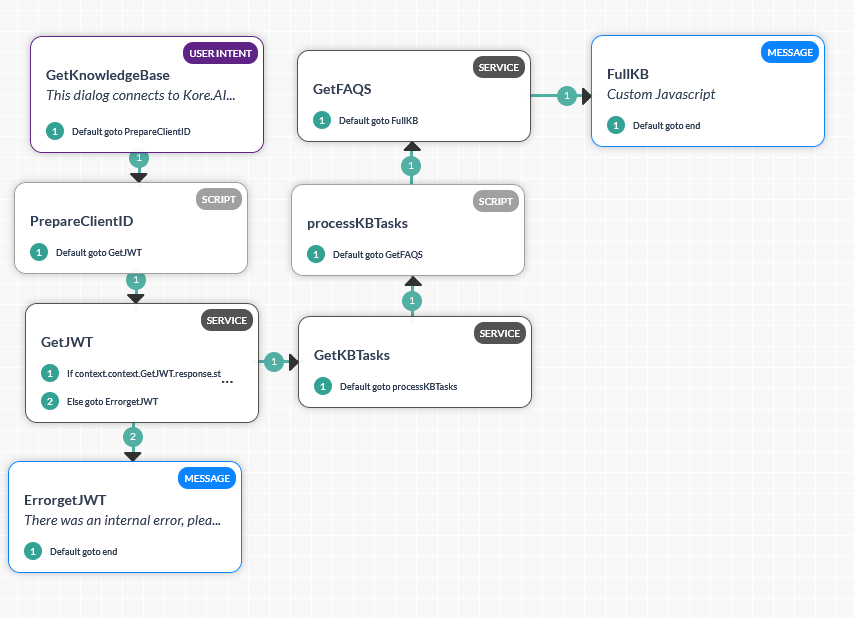
The first script is just preparing the fields botId, clientSecret, etc.
The getJWT service is contacting our internal webservice where we implement the JWT.
then, we get the list of knowledge tasks (using this api)
The answer to that web service is the taxonomy of your knowledge base.
The script process KBTasks is getting the following data:
- ktId, the knowledge Task id from the field _id
- nodeId, the identifier of the parent node you want to obtain all child elements.
This is the code from the script:
var kb = context.GetKBTasks.response.body[0].taxonomy;
context.ktId = context.GetKBTasks.response.body[0]["_id"];
context.nodeId = null;
for (var i in kb){
if (kb[i].label === context.topic){
context.nodeId = kb[i].nodeId;
break;
}
}
Finally, we invoke the getFAQS api to fetch the questions and answers of the knowledge base.
To show the questions to the end user, we are using the FullKB message node, with the following script:
var questions = [];
for (var i in context.GetFAQS.response.body.faqs){
questions.push(context.GetFAQS.response.body.faqs[i].questionPayload.question);
}
//context.GetFAQS.response.faqs[i].questionPayload.question
print(JSON.stringify(questions));